Advanced console log and other console tools in JavaScript
Logging to the console is an essential part of debugging in JavaScript. In this article, I’d like to show you some tricks and tips to make your JavaScript console log more productive and efficient.
Contents of the article:
console.log
Arguments
Most know about the console.log()
method, it outputs passed argument to the browser console.
console.log('Test')
// Test
But you can pass multiple arguments to this method:
console.log('Test', '. Hello ', 'World!')
// Test. Hello World!
Argument types can be different, that’s quite handy when you need to specify what you’re logging:
const userName = 'Jon Doe'
console.log('userName: ', userName)
// userName: Jon Doe
This way you’ll know the variable name you’re logging.
You can also log a variable as an object property by wrapping it into curly braces:
const userName = 'Jon Doe'
console.log({ userName })
// { userName: 'Jon Doe' }
This approach is useful when you need to log multiple variables or items to the console.
If you don’t have a variable name defined but the object instead, and you want to log a specific property like in the example above you can define the property inside the object you’re logging:
const user = {
name: 'Jon Doe'
}
console.log({ userName: user.name })
// { userName: 'Jon Doe' }
Also, you can use the spread operator to log the contents of the array on one line:
const user = {
name: 'Jon Doe'
}
const userData = [user, 'foo', 'bar']
console.log(...userData)
// {name: 'Jon Doe'} 'foo' 'bar'
NOTE: To log objects and arrays you can use the console.table
method listed below.
Formatting
The console.log()
method also allows you to add some styling to your messages. To do that you need to add a %c
specifier to your message and pass CSS styling as an additional parameter to the log()
method.
console.log('%c console.log is awesome', 'color: green; font-size: 16px');

console.table
Use console.table()
to display object or array data as a table.
const user = {
name: 'Jon Doe',
age: 42
}
const fruits = ['banana', 'apple', 'kiwi']
console.table(user)
console.table(fruits)
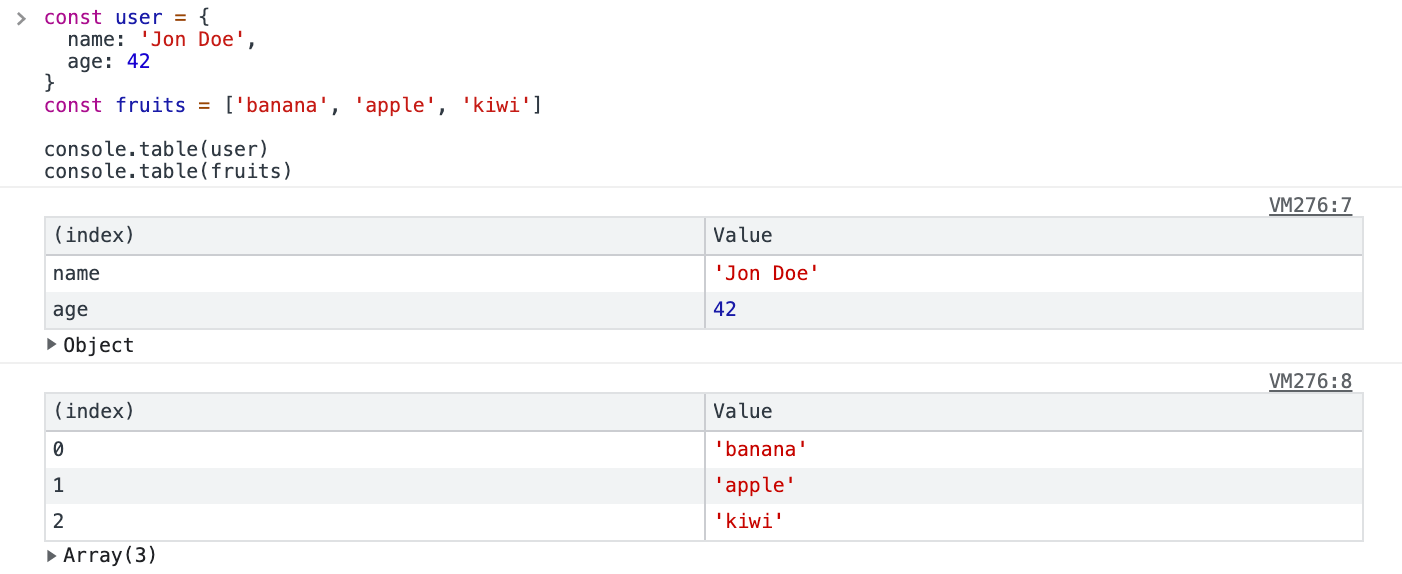
console.trace
A handy method to log to console the stack trace. It will show you the function call path up until your console.trace()
call. Quite useful during the debug process to instantly show which functions gets called.
function toggleClass(el, className) {
el.classList.toggle(className);
console.trace('toggleCLass')
}
function handleClick(e){
toggleClass(e.currentTarget, 'active')
}
const heading = document.getElementById('main-heading')
heading.addEventListener('click', handleClick)
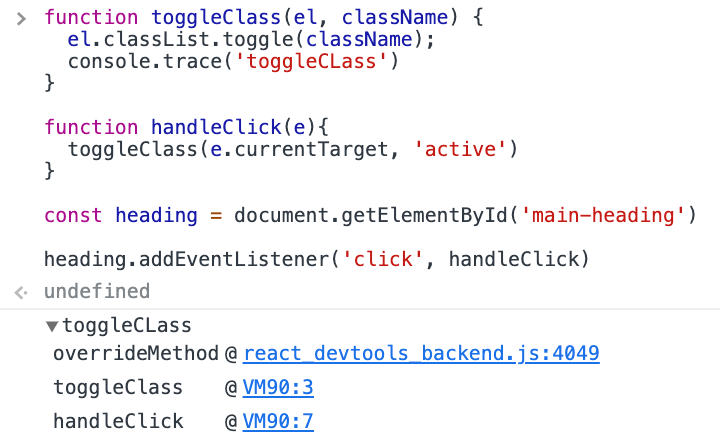
console.count
A method acts as a counter and is used to output the number of times the particular count()
method has been called.
You can pass a string (label) as an argument and it will output like a regular console.log
with a number next to it. If no argument is specified then the default label will be shown.
function handleClick(){
console.count('click count')
}
const heading = document.getElementById('main-heading')
heading.addEventListener('click', handleClick)
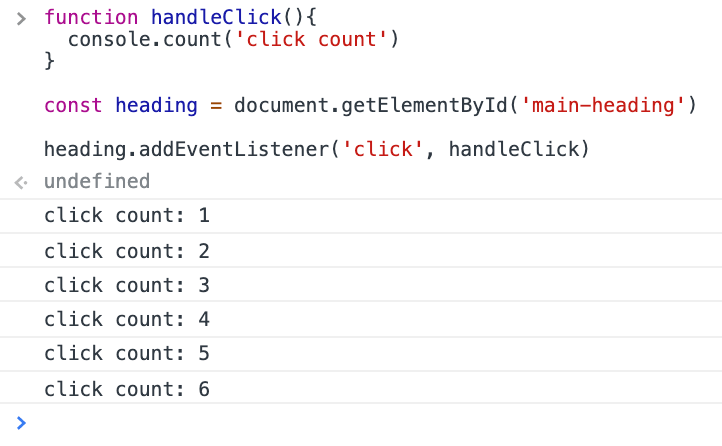
This method is useful when checking the number of times a certain function gets called, like the event handler.