How to get the current date in JavaScript (Time, Day, Month, Year)
To get the current date in JavaScript you’ll only need the Vanilla JavaScript Date API. All the information about the current date can be accessed in one line of code in various formats.
You’ll need to create a new Date object using the Date()
constructor to get the current date. The Date
constructor is supported in all browsers. Instantiate it without any parameters:
“When no parameters are provided, the newly-created Date object represents the current date and time as of the time of instantiation.”
- MDN
const currentDate = new Date()

Get Current Day
Get current week day
To get the current weekday use the getDay()
method on the currentDate
object. The return value is an integer number.
const currentDate = new Date()
currentDate.getDay()
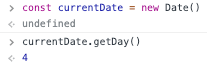
NOTE: The weekday index starts from 0, and the 0 represents Sunday.
Get current week day name
If you want to get the name of the weekday use the toLocaleString()
method with properties. The return value is a string with a language-sensitive representation of this date.
const currentDate = new Date()
currentDate.toLocaleString('en-US', { weekday: 'long' })

The first argument is the locale
which represents the language. The second is the options
object, where you can set the weekday property with a value of long.
Get current day of the month
To get the day of the month use the getDate()
method. The return value is an integer number.
const currentDate = new Date()
currentDate.getDate()
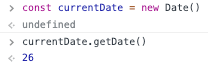
Get Current Month
Get current month number
To get the current month use the getMonth()
method. The return value is an integer number.
NOTE: Month index starts from 0, and 0 represents January.
const currentDate = new Date()
currentDate.getMonth()
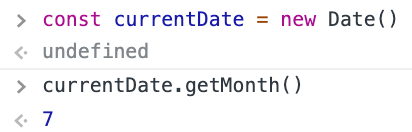
Get current month name
To get the name of the month use the toLocaleString()
method with properties. The return value is a string with a language-sensitive representation of this date.
const currentDate = new Date()
currentDate.toLocaleString('en-US', { month: 'long' })

The first argument is the locale
which represents the language. The second is the options
object, where you can set the month property with a value of long.
Get Current Year
To get the current year use the getFullYear()
method. The return value is an integer number.
const currentDate = new Date()
currentDate.getFullYear()
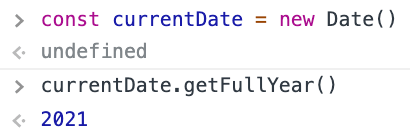
Get Current Time
To get current time you can use one of the following methods.
Get current hours
To get current hours use the getHours()
method. The return value is an integer number.
const currentDate = new Date()
currentDate.getHours()
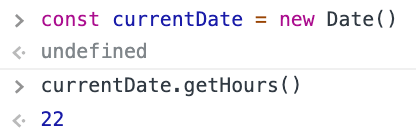
Get current minutes
To get current minutes use the getMinutes()
method. The return value is an integer number.
const currentDate = new Date()
currentDate.getMinutes()
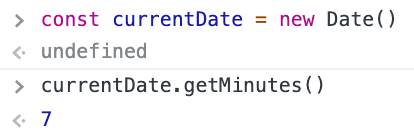
Get current seconds
To get current seconds use the getSeconds()
method. The return value is an integer number.
const currentDate = new Date()
currentDate.getSeconds()
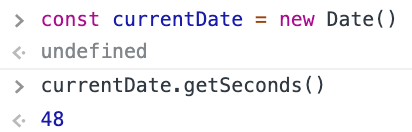
Get current time in a specific format
To get the current time in a specific time format (AM/PM or 24H) use the toLocaleTimeString()
method with properties. The return value is a string in a specific format.
const currentDate = new Date()
currentDate.toLocaleTimeString('en-US', { hour12: false })
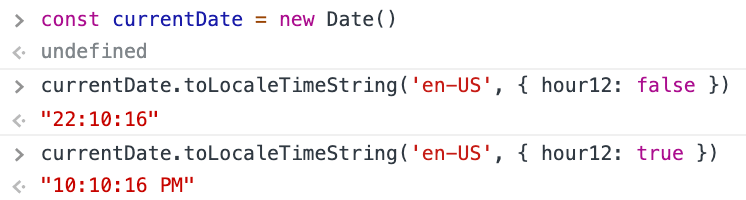
The first argument is the locale
which represents the language. The second is the options
object, where you can set the desired time format.
Get Current Date
Finally to get the current date in a string format including weekday, month, month day, and a year, use the toLocaleString()
method with parameters including the options object containing all of these values.
const currentDate = new Date()
const options = { weekday: 'long', year: 'numeric', month: 'long', day: 'numeric' };
currentDate.toLocaleString('en-US', options)

Demo
I’ve made a small tool to get any date from the Date
object. You can modify parameters and get ready to use JavaScript snippet.
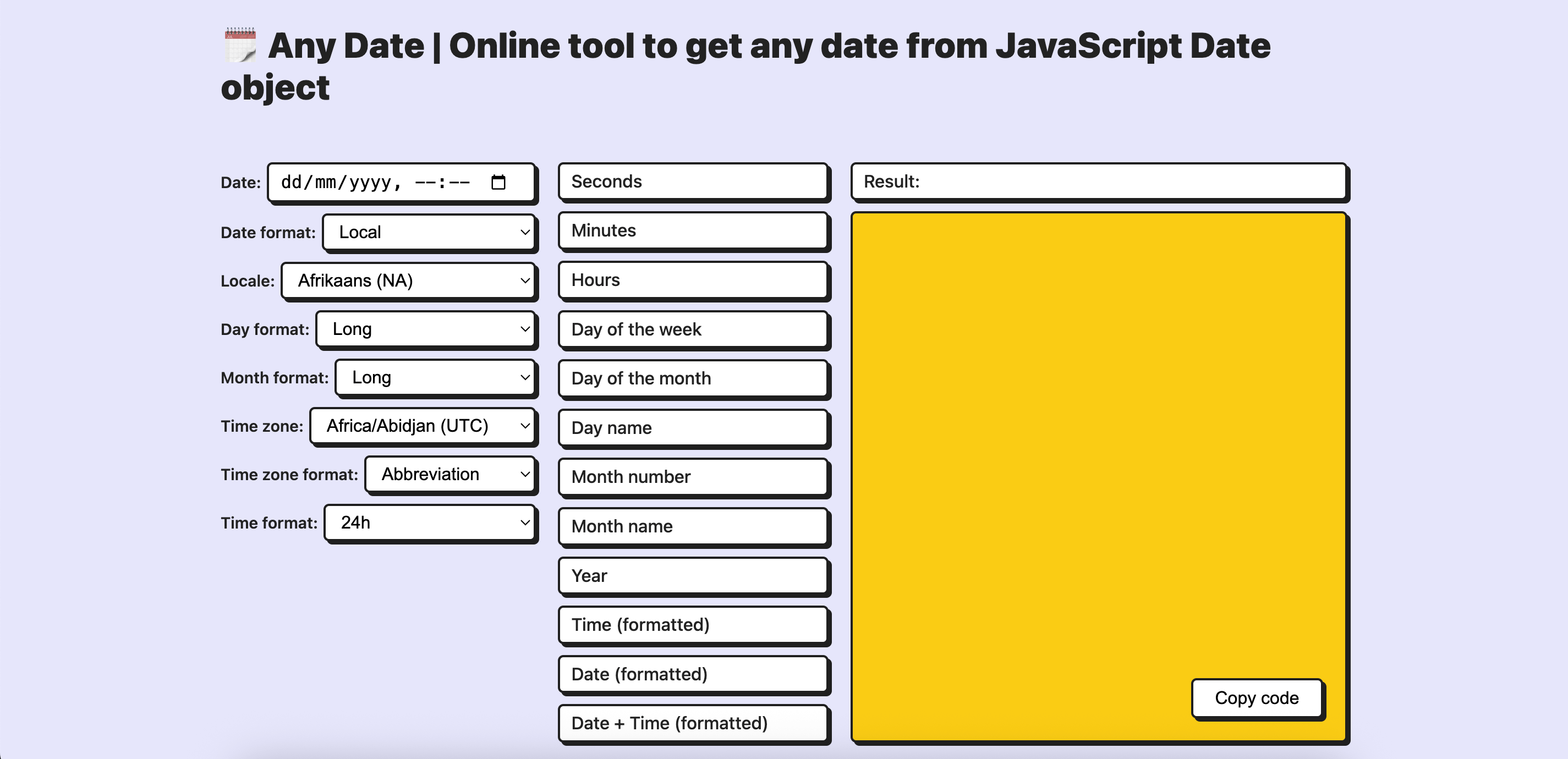