4 Ways To Get the Width and Height of an Element with Vanilla JavaScript
This guide will explain to you how to get the width and height of an HTML element with plain Vanilla JavaScript.
The contents of the article:
- The Box Model;
- offsetHeight and offsetWidth properties;
- clientHeight and clientWidth properties;
- getBoundingClientRect() method;
- getComputedStyle() method.
The Box Model
Before we move forward a small explanation about what is the Box Model and how it affects element dimensions (width and height).
You can think of an HTML element as a box. This box consists of several parts:
Every box is composed of four parts (or areas), defined by their respective edges: the content edge, padding edge, border edge, and margin edge.
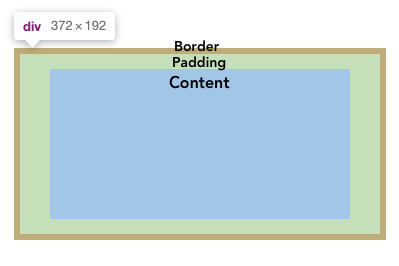
Depending on what CSS box-sizing
property is applied to the current element the element height and width can vary.
The
box-sizing
CSS property sets how the total width and height of an element is calculated.
By default, every element’s box-sizing
property is set to the content-box.
This means that if you set an element’s width to 100px
, then the element’s content box will be 100px
wide. And the width of any border or padding will be added to the final rendered width, making the element wider than 100px
.
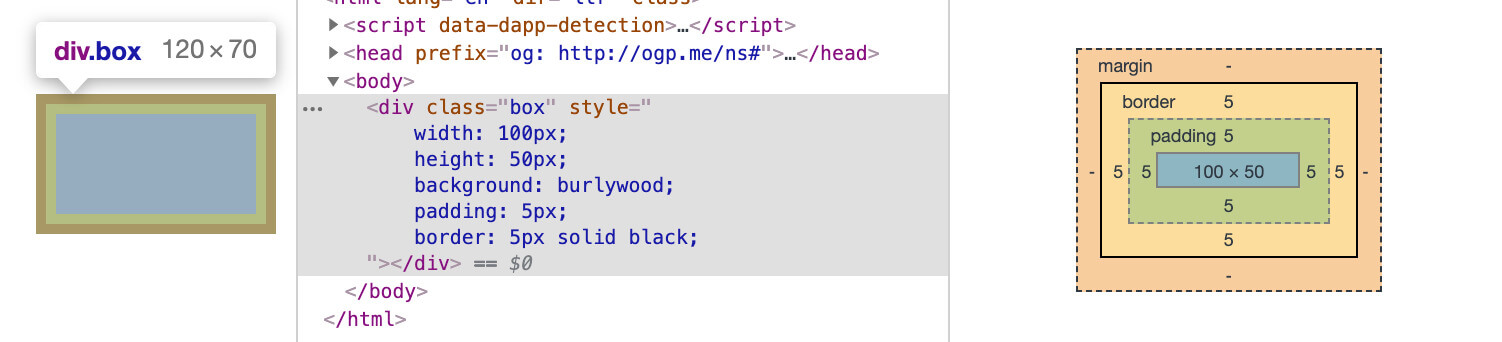
The border-box
on the other hand, tells the browser to account for any border
and padding
in the values, you specify for an element’s width
and height
. If you set an element’s width to 100px
, that 100px
will include any border
or padding
you’ve added.
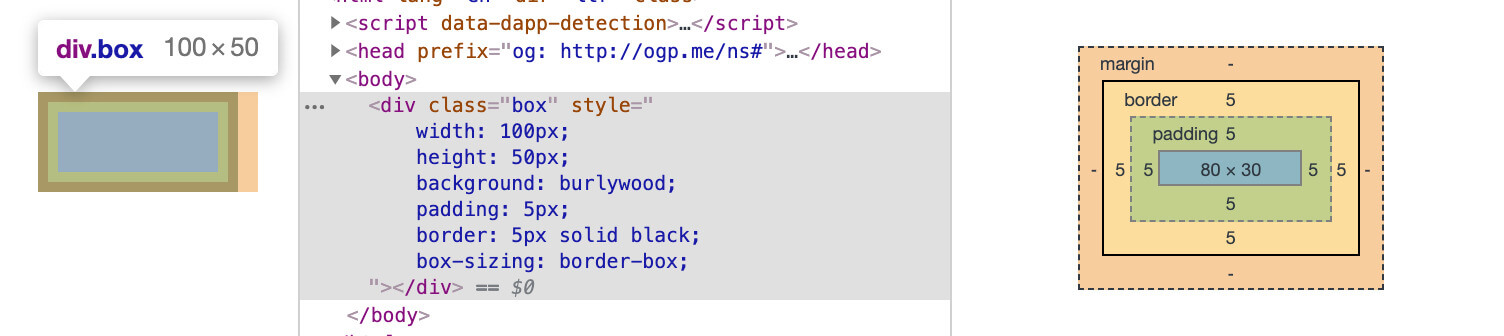
You should keep in mind what border-box
property is set to an HTML element when getting its width and height, as it may produce some unexpected results.
Moving on to how to actually get the width and height of an element with Vanilla JavaScript.
You can use one of the following Vanilla JavaScript properties and methods depending on your needs. Each one of these approaches has its own unique trait and could be used in different situations.
1. offsetHeight and offsetWidth properties
offsetHeight
and offsetWidth
properties will get the height and width of an element, including paddings and borders. The returned value is an integer and read-only.
const element = document.querySelector('.element');
element.offsetWidth;
// integer value of element’s content width + horizontal paddings and borders (if any)
element.offsetHeight;
// integer value of element’s content height + vertical paddings and borders (if any)
2. clientHeight and clientWidth properties
clientHeight
and clientWidth
properties will get the height and width of an element, including paddings but excluding borders. The returned value is an integer and read-only.
const element = document.querySelector('.element');
element.clientWidth;
// integer value of element’s content width + horizontal paddings (if any)
element.clientHeight;
// integer value of element’s content height + vertical paddings (if any)
NOTE: These properties will round the value to an integer.
3. getBoundingClientRect() method
The getBoundingClientRect()
method returns an object holding the size of an element and its position relative to the viewport. To get the width and height of the element you should use the corresponding property names.
The width
and height
properties of the getBoundingClientRect()
method will return the value based on the CSS box-sizing
property of the element. E.g. if the box-sizing
is set to border-box
, then the width
and height
will include paddings and borders.
const element = document.querySelector('.element');
element.getBoundingClientRect().width;
// integer value of element’s width (the width is calculated based on box-sizing property value)
element.getBoundingClientRect().height;
// integer value of element’s height (the height is calculated based on box-sizing property value)
4. getComputedStyle() method
The global getComputedStyle()
method returns an object containing the values of all CSS properties of an element. Each CSS value is accessible through a corresponding property and is of a string type.
Just like the method above the width
and height
properties of the getComputedStyle()
method will return the value based on the CSS box-sizing
property of the element. E.g. if the box-sizing
is set to border-box
, then the width
and height
will include paddings and borders.
const element = document.querySelector('.element');
window.getComputedStyle(element).width;
// string value of element’s content width with pixel units attached
window.getComputedStyle(element).height;
// string value of element’s content height with pixel units attached
To get the integer value just wrap it in a parseInt()
method:
parseInt(window.getComputedStyle(element).height); // integer value