How to remove HTML element from DOM with vanilla JavaScript in 4 ways
Vanilla JavaScript allows you to remove elements from DOM in several ways. In this article, I’ll show you how it can be achieved and also provide performance tests for each one.
- removeChild() method
- remove() method
- innerHTML property
- outerHTML property
- Performance tests with jsperf
1. removeChild() method
As the name states, this method will remove the child element from the selected element.
HTML:
<div id="container">
<div id="child-1"></div>
<div id="child-2"></div>
</div>
JavaScript:
const container = document.getElementById("container")
const childOne = document.getElementById("child-1")
container.removeChild(childOne)
If you’re willing to remove all children, you can use the following pattern:
while (container.firstChild) {
container.removeChild(container.firstChild);
}
This approach is fully cross-browser, read full docs on removeChild.
2. remove() method
The removeChild
method will remove the selected element from the DOM tree.
HTML:
<div id="container">
...
</div>
JavaScript:
const container = document.getElementById("container")
container.remove()
This method is not supported by Internet Explorer browser. Find out more about remove method on MDN.
3. innerHTML property
Unlike previous methods, this property’s purpose isn’t really to remove element. innerHTML
property will get or set the HTML markup contained within the element.
But you can utilize this property to “remove” any elements within the container, by setting the value to an empty string.
HTML:
<div id="container">
<div id="child-1"></div>
<div id="child-2"></div>
</div>
JavaScript:
const container = document.getElementById("container")
container.innerHTML = ""
This property is fully cross-browser, read full docs on innerHTML.
4. outerHTML property
Similar to innerHTML
the outerHTML
property allows you to get the given element as a serialized string with all the descendant elements.
Again you can utilize this property to set the value to an empty string.
HTML:
<div id="container">
<div id="child-1"></div>
<div id="child-2"></div>
</div>
JavaScript:
const container = document.getElementById("container")
container.outerHTML = ""
This property is fully cross-browser, read full docs on outerHTML.
Performance tests with jsperf
I’ve created a test on jsperf.com to compare the performance of each of those methods.
For each test case, a container will be populated with a defined HTML code that will contain ten p
elements. All p
elements from that HTML code will be removed using one of the four ways described above.
Tested in:
- Chrome 78.0.3904 / Mac OS X 10.15.1
- Firefox 71.0.0 / Mac OS X 10.15.0
- Safari 13.0.3 / Mac OS X 10.15.1
All three browsers show that for this test case the innerHTML
is the fastest way to remove multiple HTML elements from DOM.
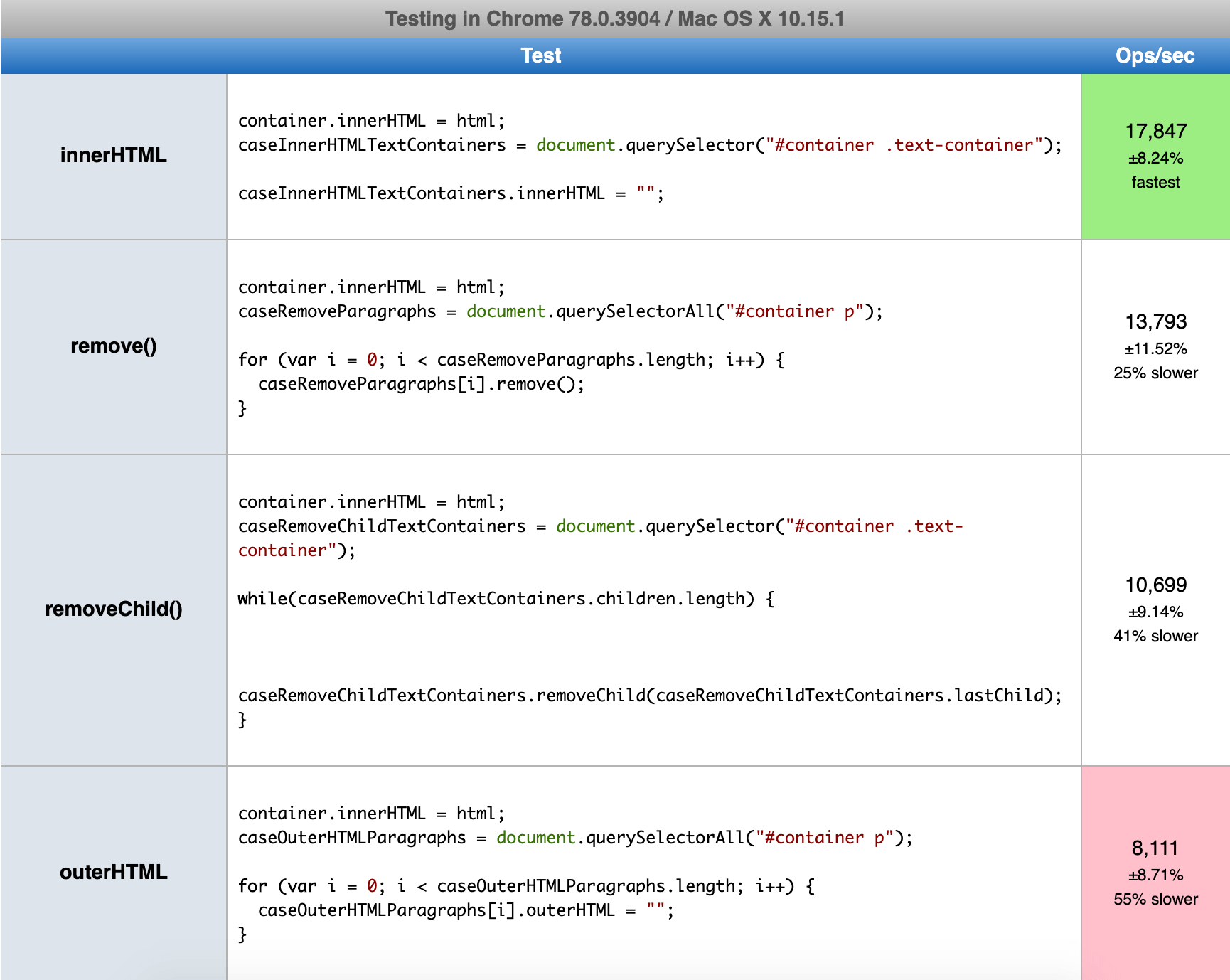
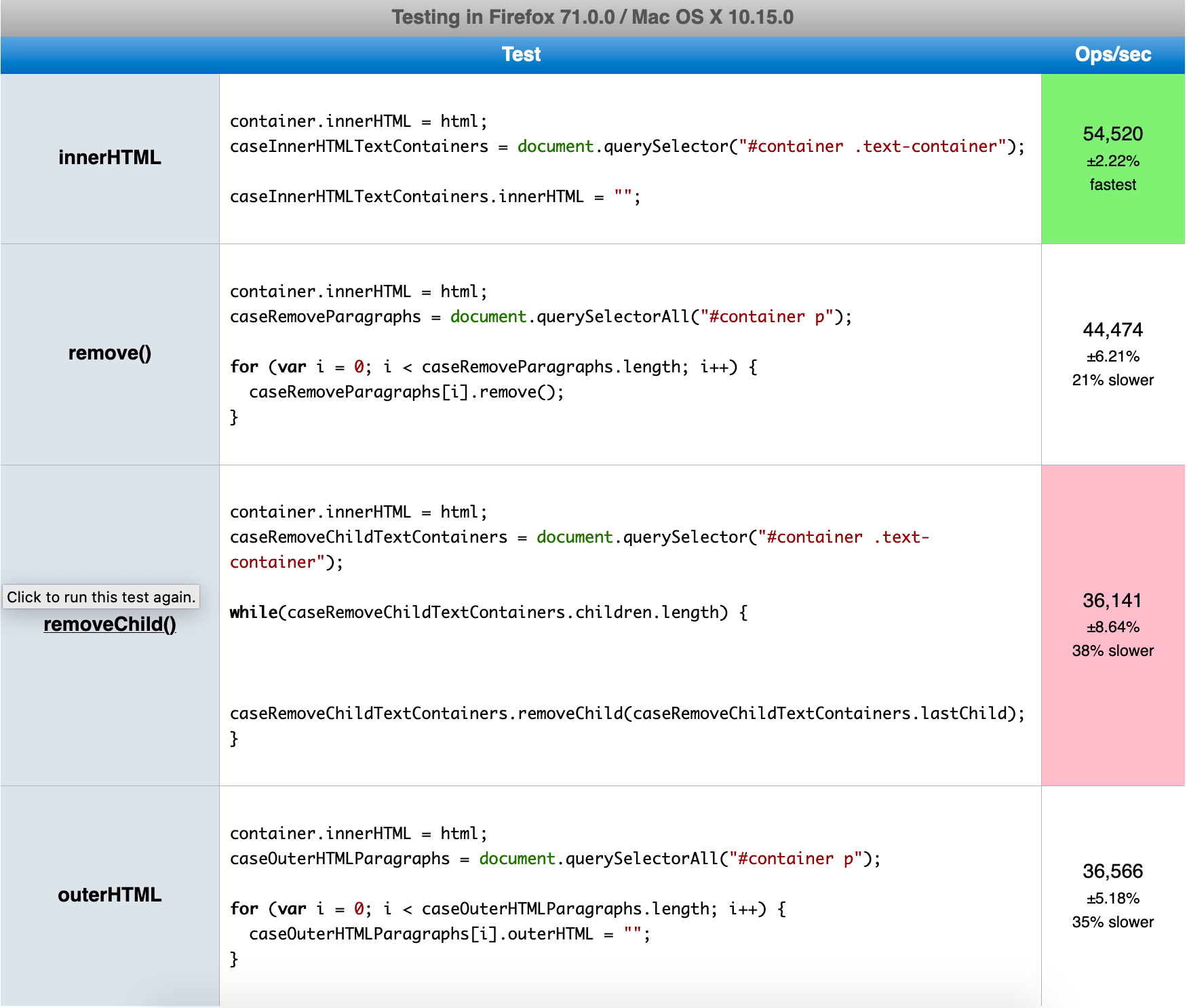
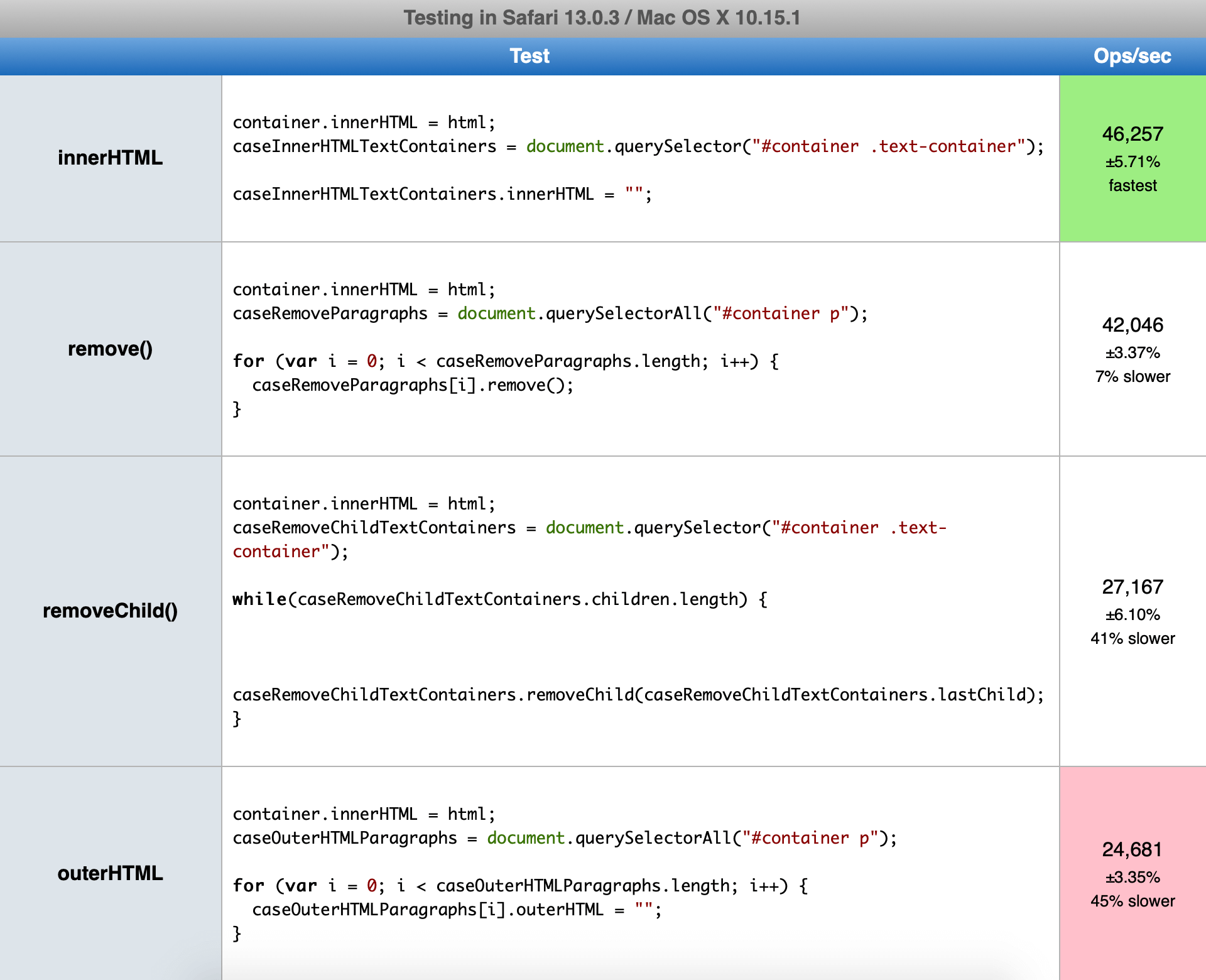