A Complete Guide How to Loop Through Object in JavaScript (+ performance tests)
There are multiple different ways how to loop through object in JavaScript. But …
NOTE: in JavaScript, there’s only one built-in way to loop through an object (for…in), all other ways are custom approaches utilizing Arrays.
That said, looping (iterating) through an object can be divided into two main approaches:
for..in
statement-
Convert the object to an Array and use different methods/loops on it
But before we move on, there’s an important detail to understand. All of the below approaches will iterate only over enumerable
object properties.
Enumerable properties are those properties whose internal enumerable flag is set to true, which is the default for properties created via simple assignment or via a property initializer
- MDN
This means that if the object’s property enumerable
flag is set to false
, then the loop will not iterate over this property.
To check an object’s property enumerable
flag you can use the getOwnPropertyDescriptor
method.
Example object.
const car = {
name: "Audi",
model: "A6",
year: 2012,
isUsed: true,
transmission: "manual"
}
Object.getOwnPropertyDescriptor(car, 'name')
// Output:
// {
// configurable: true
// enumerable: true
// value: "Audi"
// writable: true
// }
1. for…in statement
Let’s start with the built-in approach. JavaScript offers a nice way to loop over an object. It is for..in
statement. We’ll use the car
object shown above.
for (let property in car) {
console.log(`${property}: ${car[property]}`)
}
// Output:
// "name: Audi"
// "model: A6"
// "year: 2012"
// "isUsed: true"
// "transmission: manual"
As you can see you have a clear and simple construction inside which you have access to both property name and property value.
One of the drawbacks of this method that it will loop over all properties. This means that you cannot break the loop, and have to wait when it finishes.
Browser support: Supported in all browsers
2. Convert the object to an Array
The second approach is quite custom and can be divided into a few separate ways. But first, to convert the object to an Array, you can use one of three methods:
Object.keys()
- returns an array of a given object’s own enumerable property names (supported in all browsers);Object.values()
- returns an array of a given object’s own enumerable property values (some older browsers lacking support);Object.entries()
- returns an array of a given object’s own enumerable string-keyed property[key, value]
pairs (some older browsers lacking support).
Now, you can use one of the below ways to iterate over a received Array:
const carKeys = Object.keys(car)
// carKeys value:
// ["name", "model", "year", "isUsed", "transmission"]
2.1. forEach method
The first approach is to use is forEach
, one of the available Array methods.
forEach calls a provided
callback
function once for each element in an array in ascending order- MDN
For the above carKeys
Array:
carKeys.forEach((carKey, index) => {
console.log(`${index}. ${carKey}: ${car[carKey]}`)
});
// Output:
// "0. name: Audi"
// "1. model: A6"
// "2. year: 2012"
// "3. isUsed: true"
// "4. transmission: manual"
With forEach
, you are also able to get both object property name and property value for each Array entry. In addition, the callback function accepts up to four arguments: currentValue
, index
, array
, thisArg
. These arguments sometimes might be quite handy, like the index
to set a flag or place an if
statement.
Browser support: Supported in all browsers
2.2. for…of statement
The for...of
statement will iterate over iterable objects, like an Array. So with the carKeys
Array it will look like this:
for (let carKey of carKeys) {
console.log(`${carKey}: ${car[carKey]}`)
}
// Output:
// "name: Audi"
// "model: A6"
// "year: 2012"
// "isUsed: true"
// "transmission: manual"
This is very similar to the for...in
statement. The only difference is what they iterate over. And unlike the forEach
method the for...in
statement executes a code once per Array entry without any additional arguments.
Browser support: Some older browsers lacking support
2.3. for statement
The basic for
statement that you’re probably already familiar with. If not,
The for statement creates a loop that consists of three optional expressions, enclosed in parentheses and separated by semicolons, followed by a statement (usually a block statement) to be executed in the loop.
- MDN
It can be used to iterate over just about anything (except the object, like the initial car
). We can use it to loop over the carKeys
Array.
for (let i = 0; i < carKeys.length; i++) {
console.log(`${i}. ${carKeys[i]}: ${car[carKeys[i]]}`)
}
// Output:
// "0. name: Audi"
// "1. model: A6"
// "2. year: 2012"
// "3. isUsed: true"
// "4. transmission: manual"
With this approach, you also have the access to the property name, property value, and index all in one block. Another good thing about it is that you can break the loop when needed by using break
statement.
Browser support: Supported in all browsers
2.4. while loop
The last approach is to use the while
statement.
The while statement creates a loop that executes a specified statement as long as the test condition evaluates to true. The condition is evaluated before executing the statement.
- MDN
It can be used as follows:
let i = 0
while (i < carKeys.length) {
console.log(`${i}. ${carKeys[i]}: ${car[carKeys[i]]}`)
i++
}
// Output:
// "0. name: Audi"
// "1. model: A6"
// "2. year: 2012"
// "3. isUsed: true"
// "4. transmission: manual"
It is quite similar to for
statement. You have access to all the properties and values. As well as you are able to break the loop at any given point by using the break
statement.
The thing that differs it from other loops, is that you can write custom condition for the next evaluation.
Browser support: Supported in all browsers
Performance tests
For performance comparison, all of the above approaches have been run with a benchmark test at jsbench.me playground.
A benchmark test takes an object with a hundred entries and runs it for each case.
The fastest approach is the forEach
method. Performance test results can be viewed and tested at jsbench.me.
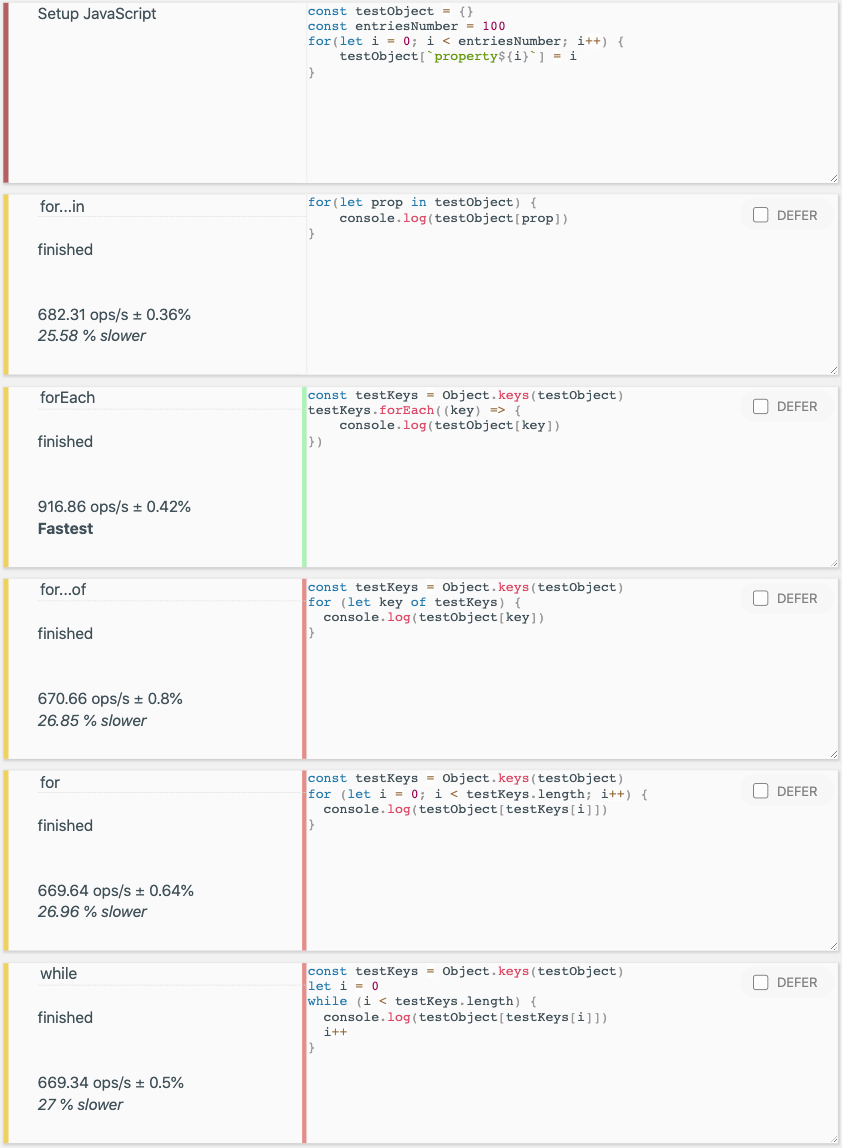